Notes on FE1008/CY1402-for, while, do while loops
This post will explain to you more on how the 3 types of loops work, similarities, differences and how to convert from one form to another--for loop, while loop, do-while loop. I provided diagrams, hopefully they can help you visualise these processes more effectively. The colours depict different stages of the operation; yellow is for initialisation, green for condition checking and when condition is satisfied, blue for incremental expressions, red for condition unsatisfied and code exiting loop. Changing from one form to another is as simple as changing the location of the initialisation, conditional and incremental expressions.
FOR LOOP
Firstly, we shall start with for loops, they are often used with arrays as the format of the for loop--with all the initialisation, conditional and incremental expression written at the same place, before the looped code--allows for very clear statement to the programmer of the number of times the code will run.
Step 1, the code enters the for loop, and initialisation happens. Following that, step 2, the program will check the condition expression and ONLY IF the condition is satisfied/true, then the code inside the curly brackets {} will be executed. Step 3 (occurs strictly after step 2 has occurred and finished) does any incremental arithmetic. The initialisation, conditional and incremental expressions are written inside the for loop.
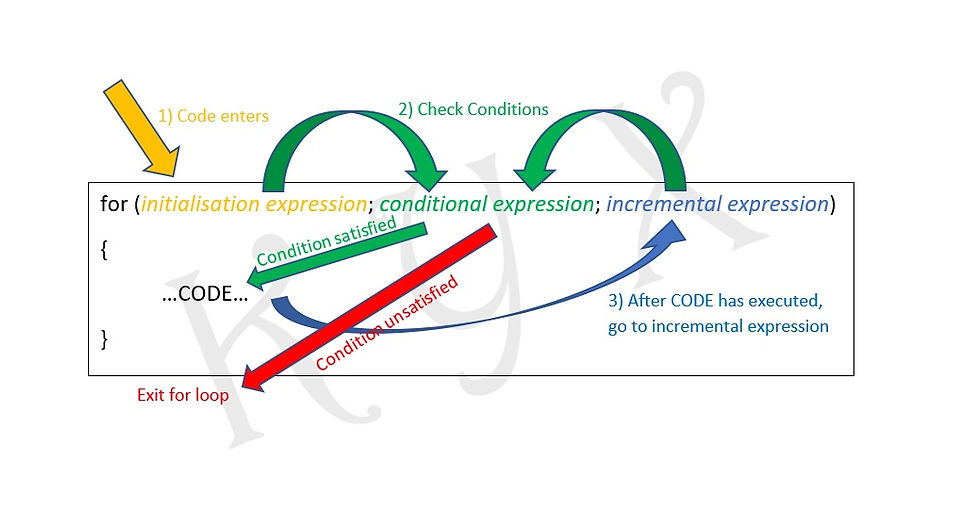
WHILE LOOP
While loop is often used in parts of the program whereby you need it to run those parts multiple times, usually looped for a infinite number of times, until a special break statement is reached.
The first initialisation step is OUTSIDE of the while loop. Then the code enters the while loop, and it first checks the conditional expression to see if it is true, the code inside the curly brackets will ONLY EXECUTES IF the condition is satisfied/true. If there is a need for incremental expression, put them in the curly braces together with the code that is going to be looped.
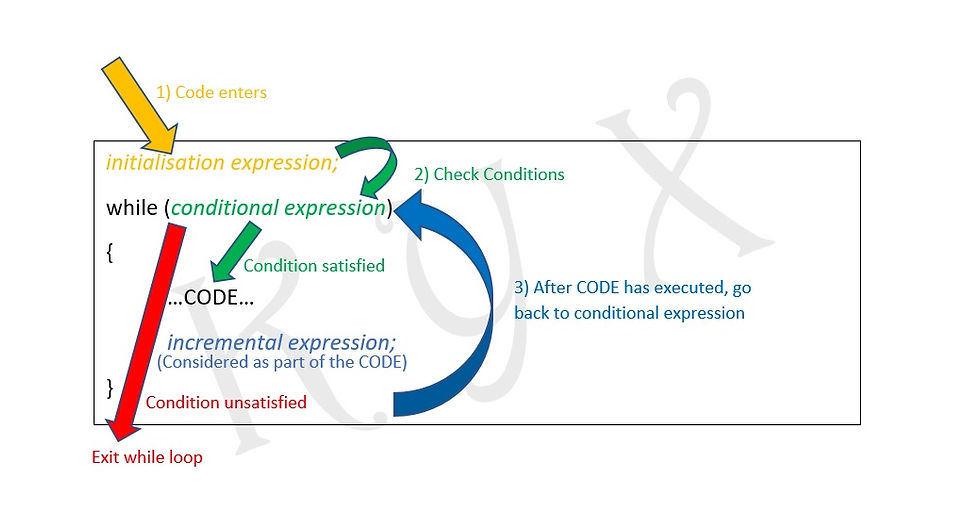
DO-WHILE LOOP
Lastly, do-while loop is very useful for asking for inputs because the code would run once before checking the condition. It can ask the user for input and then check if the user's input is valid before proceeding with the other code. If the user's input is invalid, the program will ask the user for input again until a valid input is given.
Similar to the while loop, the initialisation expression is OUTSIDE of the loop. Incremental expression has to been written together with the looped code, within the curly braces. However, the main difference compared with while loop is that WHILE LOOP is more of a CHECK-THEN-DO while DO-WHILE LOOP is more of a DO-THEN-CHECK. It is because do-while loop runs the looped code at least once before checking the conditions. An important note on this is that the looped code runs the first time regardless of whether the conditional expression is true or false. Only after looped code has been executed once, the condition will be checked, and if the condition is satisfied, the control goes back to the top of the looped code.
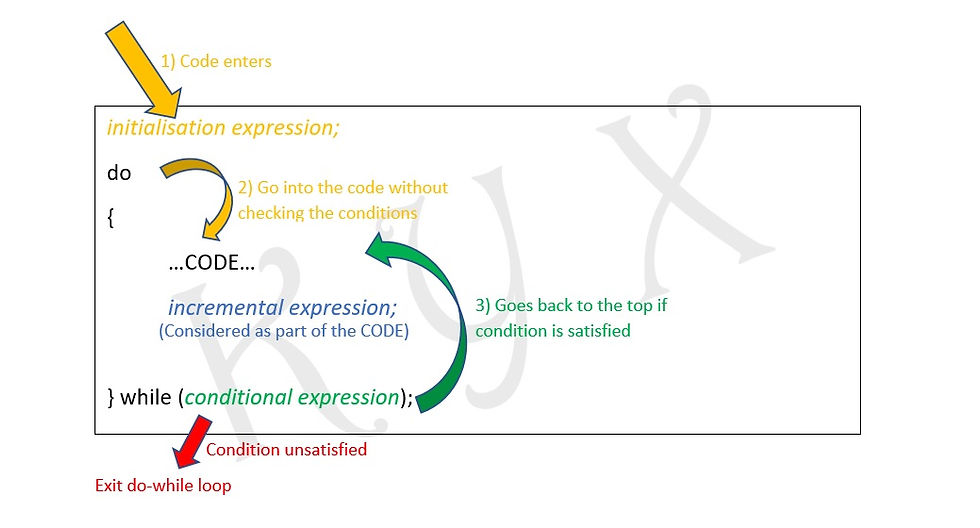
An illustration on the conversion
For example, we have the following while loop code:
i = 11; // initialisation expressions
j = 0;
while(i > 0) // Conditional expression (i > 0)
{
j++; // incremental expression that is part of the looped code
printf("Loop %d\n", j); // Looped Code
i--; // incremental expression that is also part of the looped code
}
*Special attention needs to be paid to j++; as it is before the looped code while i--; is after the looped code. This means that the value of j was adjusted before the looped code used it.
Change to For loop
I am going to show you one variation of the for loop, a for loop whereby I adjust the initial values of j (j = 1) so that I can just place j++ and i-- as part of the for loop.
for(i = 11, j = 1; i > 0; j++,i--)
{
printf("Loop %d\n", j); // Looped Code
}
Alternatively, you can treat any incremental statements before and during the code as part of the looped code in the for loop (j++ in this context). Any incremental statements after the looped code will be part of the incremental expression in the for loop (i-- in this context). In this way, there is no need for you to adjust the starting values of i and j.
for(i = 11, j = 0; i > 0; i--)
{
j++; // Considered as part of Looped Code
printf("Loop %d\n", j); // Looped Code
}
Change to Do-While Loop
Since do-while runs through the looped code once before checking the conditions, we need to be aware of what we put as the initial starting conditions and the conditions to check for. The conditions happen to be same as the while loop, but do note that this might not always be the case.
i = 11; // initialisation expressions
j = 0;
do
{
j++; // incremental expression that is part of the looped code
printf("Loop %d\n", j); // Looped Code
i--; // incremental expression that is part of the looped code
} while(i > 0);
In summary, these three types of loops can be interchanged easily by identifying the initialisation, conditional and incremental expression and placing them in the correct part of the code. Practice more to get the hang of it, computing/coding in general requires you to practice writing the code too (either in an editor or on paper) so that you go through the whole program step-by-step and become familiar with the code structures and logics.
That's all for the topic on for, while and do-while loops. If you have any doubts, opinions or suggestions, feel free to leave them in forum section or email me @ KYX@outlook.sg. Thanks~